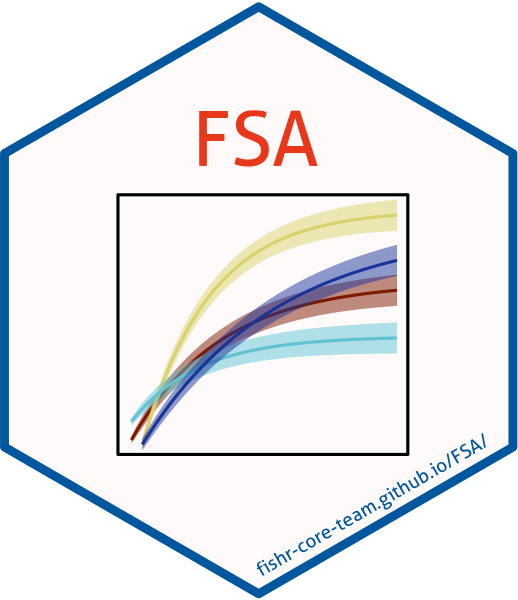
Creates a vector of Gabelhouse lengths for each species in an entire data frame.
Source:R/psdAdd.R
psdAdd.Rd
Creates a vector of the Gabelhouse lengths specific to a species for all individuals in an entire data frame.
Usage
psdAdd(len, ...)
# S3 method for default
psdAdd(
len,
species,
units = c("mm", "cm", "in"),
use.names = TRUE,
addSpec = NULL,
addLens = NULL,
verbose = TRUE,
...
)
# S3 method for formula
psdAdd(
len,
data = NULL,
units = c("mm", "cm", "in"),
use.names = TRUE,
addSpec = NULL,
addLens = NULL,
verbose = TRUE,
...
)
Arguments
- len
A numeric vector that contains lengths measurements or a formula of the form
len~spec
where “len” generically represents the length variable and “spec” generically represents the species variable. Note that this formula can only contain two variables and must have the length variable on the left-hand-side and the species variable on the right-hand-side.- ...
Not used.
- species
A character or factor vector that contains the species names. Ignored if
len
is a formula.- units
A string that indicates the type of units used for the lengths. Choices are
mm
for millimeters (DEFAULT),cm
for centimeters, andin
for inches.- use.names
A logical that indicates whether the vector returned is numeric (
=FALSE
) or string (=TRUE
; default) representations of the Gabelhouse lengths. See details.- addSpec
A character vector of species names for which
addLens
will be provided.- addLens
A numeric vector of lengths that should be used in addition to the Gabelhouse lengths for the species in
addSpec
. See examples.- verbose
A logical that indicates whether detailed messages about species without Gabelhouse lengths or with no recorded values should be printed or not.
- data
A data.frame that minimally contains the length measurements and species names if
len
is a formula.
Details
This computes a vector that contains the Gabelhouse lengths specific to each species for all individuals in an entire data frame. The vector can be appended to an existing data.frame to create a variable that contains the Gabelhouse lengths for each individual. The Gabelhouse length value will be NA
for each individual for which Gabelhouse length definitions do not exist in PSDlit
. Species names in the data.frame must be the same as those used in PSDlit
. See the examples for one method for changing species names to something that this function will recognize.
Individuals shorter than “stock” length will be listed as substock
if use.names=TRUE
or 0
if use.names=FALSE
.
Additional lengths to be used for a species may be included by giving a vector of species names in addSpec
and a corresponding vector of additional lengths in addLens
. Note, however, that use.names
will be reset to FALSE
if addSpec
and addLens
are specified, as there is no way to order the names for all species when additional lengths are used.
References
Ogle, D.H. 2016. Introductory Fisheries Analyses with R. Chapman & Hall/CRC, Boca Raton, FL.
Guy, C.S., R.M. Neumann, and D.W. Willis. 2006. New terminology for proportional stock density (PSD) and relative stock density (RSD): proportional size structure (PSS). Fisheries 31:86-87. [Was (is?) from http://pubstorage.sdstate.edu/wfs/415-F.pdf.]
Guy, C.S., R.M. Neumann, D.W. Willis, and R.O. Anderson. 2006. Proportional size distribution (PSD): A further refinement of population size structure index terminology. Fisheries 32:348. [Was (is?) from http://pubstorage.sdstate.edu/wfs/450-F.pdf.]
Willis, D.W., B.R. Murphy, and C.S. Guy. 1993. Stock density indices: development, use, and limitations. Reviews in Fisheries Science 1:203-222. [Was (is?) from http://web1.cnre.vt.edu/murphybr/web/Readings/Willis%20et%20al.pdf.]
Author
Derek H. Ogle, DerekOgle51@gmail.com
Examples
## Create random data for three species
# only for repeatability
set.seed(345234534)
dbg <- data.frame(species=factor(rep(c("Bluegill"),30)),
tl=round(rnorm(30,130,50),0))
dbg$wt <- round(4.23e-06*dbg$tl^3.316+rnorm(30,0,10),1)
dlb <- data.frame(species=factor(rep(c("Largemouth Bass"),30)),
tl=round(rnorm(30,350,60),0))
dlb$wt <- round(2.96e-06*dlb$tl^3.273+rnorm(30,0,60),1)
dbt <- data.frame(species=factor(rep(c("Bluefin Tuna"),30)),
tl=round(rnorm(30,1900,300),0))
dbt$wt <- round(4.5e-05*dbt$tl^2.8+rnorm(30,0,6000),1)
df <- rbind(dbg,dlb,dbt)
str(df)
#> 'data.frame': 90 obs. of 3 variables:
#> $ species: Factor w/ 3 levels "Bluegill","Largemouth Bass",..: 1 1 1 1 1 1 1 1 1 1 ...
#> $ tl : num 42 73 152 184 167 94 138 169 86 157 ...
#> $ wt : num -12.5 4.8 86.6 134.4 91.9 ...
## Examples (non-dplyr)
# Add variable using category names -- formula notation
df$PSD <- psdAdd(tl~species,data=df)
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
head(df)
#> species tl wt PSD
#> 1 Bluegill 42 -12.5 substock
#> 2 Bluegill 73 4.8 substock
#> 3 Bluegill 152 86.6 quality
#> 4 Bluegill 184 134.4 quality
#> 5 Bluegill 167 91.9 quality
#> 6 Bluegill 94 38.3 stock
# Add variable using category names -- non-formula notation
df$PSD1 <- psdAdd(df$tl,df$species)
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
head(df)
#> species tl wt PSD PSD1
#> 1 Bluegill 42 -12.5 substock substock
#> 2 Bluegill 73 4.8 substock substock
#> 3 Bluegill 152 86.6 quality quality
#> 4 Bluegill 184 134.4 quality quality
#> 5 Bluegill 167 91.9 quality quality
#> 6 Bluegill 94 38.3 stock stock
# Add variable using length values as names
df$PSD2 <- psdAdd(tl~species,data=df,use.names=FALSE)
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
head(df)
#> species tl wt PSD PSD1 PSD2
#> 1 Bluegill 42 -12.5 substock substock 0
#> 2 Bluegill 73 4.8 substock substock 0
#> 3 Bluegill 152 86.6 quality quality 150
#> 4 Bluegill 184 134.4 quality quality 150
#> 5 Bluegill 167 91.9 quality quality 150
#> 6 Bluegill 94 38.3 stock stock 80
# Add additional length and name for Bluegill
df$PSD3 <- psdAdd(tl~species,data=df,addSpec="Bluegill",addLens=175)
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
head(df)
#> species tl wt PSD PSD1 PSD2 PSD3
#> 1 Bluegill 42 -12.5 substock substock 0 0
#> 2 Bluegill 73 4.8 substock substock 0 0
#> 3 Bluegill 152 86.6 quality quality 150 150
#> 4 Bluegill 184 134.4 quality quality 150 175
#> 5 Bluegill 167 91.9 quality quality 150 150
#> 6 Bluegill 94 38.3 stock stock 80 80
# Add add'l lengths and names for Bluegill and Largemouth Bass from a data.frame
addls <- data.frame(species=c("Bluegill","Largemouth Bass","Largemouth Bass"),
lens=c(175,254,356))
df$psd4 <- psdAdd(tl~species,data=df,addSpec=addls$species,addLens=addls$lens)
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
head(df)
#> species tl wt PSD PSD1 PSD2 PSD3 psd4
#> 1 Bluegill 42 -12.5 substock substock 0 0 0
#> 2 Bluegill 73 4.8 substock substock 0 0 0
#> 3 Bluegill 152 86.6 quality quality 150 150 150
#> 4 Bluegill 184 134.4 quality quality 150 175 175
#> 5 Bluegill 167 91.9 quality quality 150 150 150
#> 6 Bluegill 94 38.3 stock stock 80 80 80
## All of the above but using dplyr
if (require(dplyr)) {
df <- df %>%
mutate(PSD1A=psdAdd(tl,species)) %>%
mutate(PSD2A=psdAdd(tl,species,use.names=FALSE)) %>%
mutate(psd3a=psdAdd(tl,species,addSpec="Bluegill",addLens=175)) %>%
mutate(psd4a=psdAdd(tl,species,addSpec=addls$species,addLens=addls$lens))
}
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
#> No known Gabelhouse (PSD) lengths for: Bluefin Tuna
df
#> species tl wt PSD PSD1 PSD2 PSD3 psd4 PSD1A
#> 1 Bluegill 42 -12.5 substock substock 0 0 0 substock
#> 2 Bluegill 73 4.8 substock substock 0 0 0 substock
#> 3 Bluegill 152 86.6 quality quality 150 150 150 quality
#> 4 Bluegill 184 134.4 quality quality 150 175 175 quality
#> 5 Bluegill 167 91.9 quality quality 150 150 150 quality
#> 6 Bluegill 94 38.3 stock stock 80 80 80 stock
#> 7 Bluegill 138 62.5 stock stock 80 80 80 stock
#> 8 Bluegill 169 93.9 quality quality 150 150 150 quality
#> 9 Bluegill 86 20.7 stock stock 80 80 80 stock
#> 10 Bluegill 157 79.1 quality quality 150 150 150 quality
#> 11 Bluegill 123 36.2 stock stock 80 80 80 stock
#> 12 Bluegill 210 207.8 preferred preferred 200 200 200 preferred
#> 13 Bluegill 150 77.2 quality quality 150 150 150 quality
#> 14 Bluegill 124 27.6 stock stock 80 80 80 stock
#> 15 Bluegill 116 31.1 stock stock 80 80 80 stock
#> 16 Bluegill 139 61.9 stock stock 80 80 80 stock
#> 17 Bluegill 160 72.5 quality quality 150 150 150 quality
#> 18 Bluegill 120 40.1 stock stock 80 80 80 stock
#> 19 Bluegill 155 90.6 quality quality 150 150 150 quality
#> 20 Bluegill 238 301.6 preferred preferred 200 200 200 preferred
#> 21 Bluegill 153 103.1 quality quality 150 150 150 quality
#> 22 Bluegill 32 -11.4 substock substock 0 0 0 substock
#> 23 Bluegill 151 66.8 quality quality 150 150 150 quality
#> 24 Bluegill 108 14.0 stock stock 80 80 80 stock
#> 25 Bluegill 142 58.9 stock stock 80 80 80 stock
#> 26 Bluegill 124 31.3 stock stock 80 80 80 stock
#> 27 Bluegill 109 23.3 stock stock 80 80 80 stock
#> 28 Bluegill 94 27.1 stock stock 80 80 80 stock
#> 29 Bluegill 196 162.8 quality quality 150 175 175 quality
#> 30 Bluegill 128 28.6 stock stock 80 80 80 stock
#> 31 Largemouth Bass 384 807.1 preferred preferred 380 380 380 preferred
#> 32 Largemouth Bass 383 840.2 preferred preferred 380 380 380 preferred
#> 33 Largemouth Bass 346 527.1 quality quality 300 300 300 quality
#> 34 Largemouth Bass 437 1337.9 preferred preferred 380 380 380 preferred
#> 35 Largemouth Bass 357 728.2 quality quality 300 300 356 quality
#> 36 Largemouth Bass 425 1196.0 preferred preferred 380 380 380 preferred
#> 37 Largemouth Bass 353 702.1 quality quality 300 300 300 quality
#> 38 Largemouth Bass 340 551.1 quality quality 300 300 300 quality
#> 39 Largemouth Bass 358 629.4 quality quality 300 300 356 quality
#> 40 Largemouth Bass 257 181.2 stock stock 200 200 254 stock
#> 41 Largemouth Bass 327 454.1 quality quality 300 300 300 quality
#> 42 Largemouth Bass 465 1573.9 preferred preferred 380 380 380 preferred
#> 43 Largemouth Bass 454 1481.4 preferred preferred 380 380 380 preferred
#> 44 Largemouth Bass 389 859.2 preferred preferred 380 380 380 preferred
#> 45 Largemouth Bass 336 465.6 quality quality 300 300 300 quality
#> 46 Largemouth Bass 289 393.5 stock stock 200 200 254 stock
#> 47 Largemouth Bass 288 305.1 stock stock 200 200 254 stock
#> 48 Largemouth Bass 401 1007.1 preferred preferred 380 380 380 preferred
#> 49 Largemouth Bass 321 509.4 quality quality 300 300 300 quality
#> 50 Largemouth Bass 340 581.2 quality quality 300 300 300 quality
#> 51 Largemouth Bass 308 406.4 quality quality 300 300 300 quality
#> 52 Largemouth Bass 250 179.9 stock stock 200 200 200 stock
#> 53 Largemouth Bass 380 793.7 preferred preferred 380 380 380 preferred
#> 54 Largemouth Bass 394 938.8 preferred preferred 380 380 380 preferred
#> 55 Largemouth Bass 369 755.6 quality quality 300 300 356 quality
#> 56 Largemouth Bass 344 692.6 quality quality 300 300 300 quality
#> 57 Largemouth Bass 273 208.7 stock stock 200 200 254 stock
#> 58 Largemouth Bass 386 901.4 preferred preferred 380 380 380 preferred
#> 59 Largemouth Bass 296 309.7 stock stock 200 200 254 stock
#> 60 Largemouth Bass 280 380.1 stock stock 200 200 254 stock
#> 61 Bluefin Tuna 1904 58963.4 <NA> <NA> NA NA NA <NA>
#> 62 Bluefin Tuna 1634 56007.5 <NA> <NA> NA NA NA <NA>
#> 63 Bluefin Tuna 1938 64262.2 <NA> <NA> NA NA NA <NA>
#> 64 Bluefin Tuna 2403 131188.3 <NA> <NA> NA NA NA <NA>
#> 65 Bluefin Tuna 2026 70580.7 <NA> <NA> NA NA NA <NA>
#> 66 Bluefin Tuna 2294 116401.4 <NA> <NA> NA NA NA <NA>
#> 67 Bluefin Tuna 2116 90855.2 <NA> <NA> NA NA NA <NA>
#> 68 Bluefin Tuna 991 15764.4 <NA> <NA> NA NA NA <NA>
#> 69 Bluefin Tuna 1940 61447.3 <NA> <NA> NA NA NA <NA>
#> 70 Bluefin Tuna 2129 89686.6 <NA> <NA> NA NA NA <NA>
#> 71 Bluefin Tuna 1743 55336.4 <NA> <NA> NA NA NA <NA>
#> 72 Bluefin Tuna 1773 54506.3 <NA> <NA> NA NA NA <NA>
#> 73 Bluefin Tuna 1673 46411.8 <NA> <NA> NA NA NA <NA>
#> 74 Bluefin Tuna 1864 56358.0 <NA> <NA> NA NA NA <NA>
#> 75 Bluefin Tuna 1945 76569.3 <NA> <NA> NA NA NA <NA>
#> 76 Bluefin Tuna 1623 42905.7 <NA> <NA> NA NA NA <NA>
#> 77 Bluefin Tuna 1923 60858.8 <NA> <NA> NA NA NA <NA>
#> 78 Bluefin Tuna 1831 69531.2 <NA> <NA> NA NA NA <NA>
#> 79 Bluefin Tuna 2658 175368.5 <NA> <NA> NA NA NA <NA>
#> 80 Bluefin Tuna 1788 62130.2 <NA> <NA> NA NA NA <NA>
#> 81 Bluefin Tuna 2084 91052.6 <NA> <NA> NA NA NA <NA>
#> 82 Bluefin Tuna 1770 58165.3 <NA> <NA> NA NA NA <NA>
#> 83 Bluefin Tuna 2085 88694.5 <NA> <NA> NA NA NA <NA>
#> 84 Bluefin Tuna 1521 32761.0 <NA> <NA> NA NA NA <NA>
#> 85 Bluefin Tuna 2337 126556.8 <NA> <NA> NA NA NA <NA>
#> 86 Bluefin Tuna 1716 48947.6 <NA> <NA> NA NA NA <NA>
#> 87 Bluefin Tuna 1448 26363.9 <NA> <NA> NA NA NA <NA>
#> 88 Bluefin Tuna 2356 119531.1 <NA> <NA> NA NA NA <NA>
#> 89 Bluefin Tuna 1481 34928.6 <NA> <NA> NA NA NA <NA>
#> 90 Bluefin Tuna 2007 64746.8 <NA> <NA> NA NA NA <NA>
#> PSD2A psd3a psd4a
#> 1 0 0 0
#> 2 0 0 0
#> 3 150 150 150
#> 4 150 175 175
#> 5 150 150 150
#> 6 80 80 80
#> 7 80 80 80
#> 8 150 150 150
#> 9 80 80 80
#> 10 150 150 150
#> 11 80 80 80
#> 12 200 200 200
#> 13 150 150 150
#> 14 80 80 80
#> 15 80 80 80
#> 16 80 80 80
#> 17 150 150 150
#> 18 80 80 80
#> 19 150 150 150
#> 20 200 200 200
#> 21 150 150 150
#> 22 0 0 0
#> 23 150 150 150
#> 24 80 80 80
#> 25 80 80 80
#> 26 80 80 80
#> 27 80 80 80
#> 28 80 80 80
#> 29 150 175 175
#> 30 80 80 80
#> 31 380 380 380
#> 32 380 380 380
#> 33 300 300 300
#> 34 380 380 380
#> 35 300 300 356
#> 36 380 380 380
#> 37 300 300 300
#> 38 300 300 300
#> 39 300 300 356
#> 40 200 200 254
#> 41 300 300 300
#> 42 380 380 380
#> 43 380 380 380
#> 44 380 380 380
#> 45 300 300 300
#> 46 200 200 254
#> 47 200 200 254
#> 48 380 380 380
#> 49 300 300 300
#> 50 300 300 300
#> 51 300 300 300
#> 52 200 200 200
#> 53 380 380 380
#> 54 380 380 380
#> 55 300 300 356
#> 56 300 300 300
#> 57 200 200 254
#> 58 380 380 380
#> 59 200 200 254
#> 60 200 200 254
#> 61 NA NA NA
#> 62 NA NA NA
#> 63 NA NA NA
#> 64 NA NA NA
#> 65 NA NA NA
#> 66 NA NA NA
#> 67 NA NA NA
#> 68 NA NA NA
#> 69 NA NA NA
#> 70 NA NA NA
#> 71 NA NA NA
#> 72 NA NA NA
#> 73 NA NA NA
#> 74 NA NA NA
#> 75 NA NA NA
#> 76 NA NA NA
#> 77 NA NA NA
#> 78 NA NA NA
#> 79 NA NA NA
#> 80 NA NA NA
#> 81 NA NA NA
#> 82 NA NA NA
#> 83 NA NA NA
#> 84 NA NA NA
#> 85 NA NA NA
#> 86 NA NA NA
#> 87 NA NA NA
#> 88 NA NA NA
#> 89 NA NA NA
#> 90 NA NA NA